A Little Ruby
A Snippet into Ruby Classes
When and Why Should I use classes?
One question which kept simmering in my head while working on this week's challenges was "when do you use Ruby classes?". Well a class is necessary when instantiation is required or when the functionality of the code needs top keep track of state. Think of class as a factory manufacturing more than one object. If just wanted one object you wouldn't use class. Let's go through an example of class Car.
So, How do I create a class?
It's as simple as typing "class", a capitalized name, and closing it with "end"

What's a Constructor?
When creating a class, it's most likely you will want the instances of the class to have some properties. You can do this by creating a constructor at the top of the class code which utilizes "initialize" and takes a number of arguments for the instance of class. From our Car class example we can now initialize Car make, model, and year.
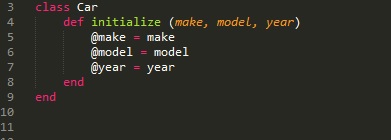
A shortcut for this would be to simply call "attr_accessor" and the instance variable as a symbol( with a colon in front ). This way you can cut down on the lines of code as well as save time.
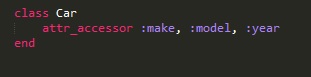
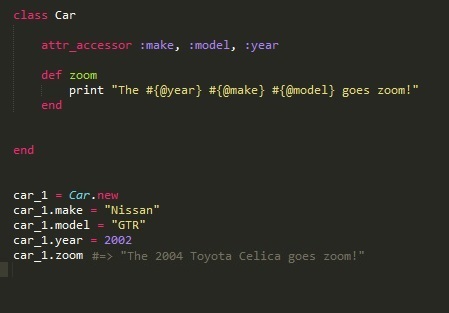
Instance Methods
Create an instance method as you would normally in Ruby by defining a method name, the code you want the method to execute, and close it.
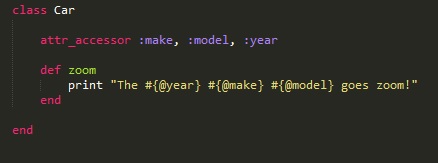
Creating Class Methods and Variables
Class methods and properties are variables and functions inaccessible to instances of the class. They are exclusive to the class itself. So if we wanted to know the number of Car instances we created we would set the count with two "@" symbols declaring it as a class variable equal to zero. To increment this variable with the number of instances we put it within our initialize method. We also have to create our class method. By calling self.count we tell Ruby to count how many instances of Car were created.
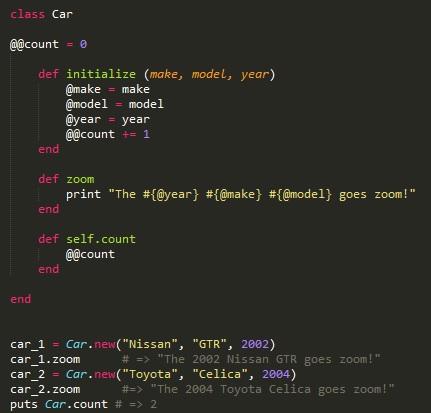
Data Structures: Hashes and Arrays
We can use hashes and arrays to store/access information in classes. Let's use our same class Car and add a nested hash. Now we want a report of owners and details about their car. We can iterate through the nested hash using "each_key" method, create an instance and access the hash using the hash name, the owner name in brackets (which is the key of the inner hash), and then we call the key within that hash "make" in square brackets. We repeat this for the properties make, model, year. Don't forget to close the iteration!
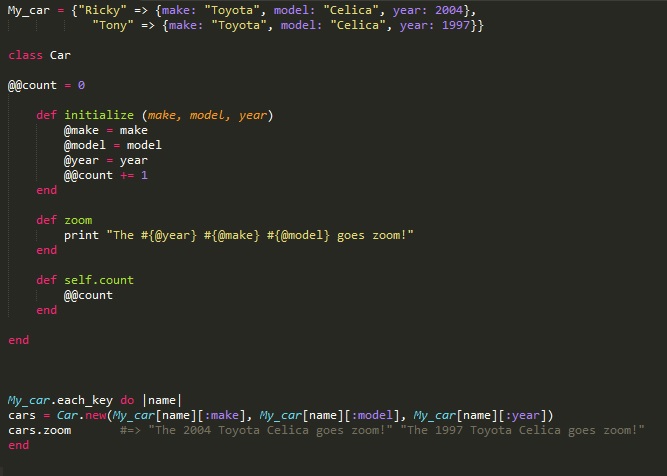
Posts
-
The Post DBC Experience
Mixed Emotions
DBC was an awesome, maybe one of a kind experience. I pushed through all 4 phases with the mantids, or the improper plural...
-
How to Ask for Help
How to Ask Good Questions
Experienced Questioning
I don't always ask questions online but when I do I try to be as specific as possible...
-
Ruby on Rails
Rails
Ruby and Rails are not the same thing. Ruby is the programming language. Rails is a Rubygem or package library which is installed via...
-
Resolving Conflicts
A Conflict I Experienced
Honestly, I cannot remember a conflict I've experienced off the top of my head that would be exciting to read or...
- My Thinking Ways How do I Think? I am a concrete sequential thinker which according to cgribben.com mean that I am persistent and product oriented. I am practical...
-
SQL Injection
What is SQL?
SQL is short for Structured Query Language. It is a programming language for querying (fancy word for accessing) and managing databases. SQL...
-
Affirmation and Stereotype Threat
My Values
Accomplishment, accountability, compassion, creativity, efficiency, family, friendships, and integrity area few values which come to mind when I recall the times in my...
-
Blocs, Procs, and Lambdas
Ruby Blocks, Procs, Lambdas
Blocks
A ruby block is the piece of code that is executed. This code can be executed using curly brackets {}...
-
Stereotype Threat
What is Stereotype Threat?
To put it simply, stereotype threat is the experience of not living or carrying out your full potential because of stereotypes....
-
A Pair of Minds
Pairing and Feedback
Pairing
Pairing isn't so bad. The peers are all nice and I haven't met someone who was disrespectful during the sessions. Despite...
-
A Little Ruby
A Snippet into Ruby Classes
When and Why Should I use classes?
One question which kept simmering in my head while working on this week's...
-
Ruby Cycle and Looping
Ruby:Enumberable#Cycle
The #cycle method uses a block for each element of an enumerable object (ranges, hash, or array) repeatedly for n times or endlessly if...
-
Today's Software Apps vs Law
Who Doesn’t Like App Efficiency?
Innovative technologies usually clash with old ways and methods. There are numerous apps out there which are constantly clashing with...
-
What is JS?
What is JavaScript and Why Should You Care?
Javascript(JS) is an object oriented computer programming language often found as a part of web browsers which...
-
CSS Positioning
Positioning in CSS
Elements on a website can be positioned behind, in front of, or next to by using CSS positioning properties: top, left, right,...
-
My Favorite Websites
My 3 Most Visited Websites
Newcelica.org
This is one of my favorite sites because I own a Celica. I do all...
-
Kitchen Vs Table
Kitchen Vs Table
DBC sounds like a buttkicker, a mental, emotional, and physical obstacle course. Honestly, it sounds like it would and should be overwhelming...